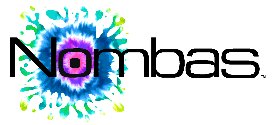
The Clib object
The Clib object contains methods for the standard C library.
This includes methods for working with files, strings, A good
portion of the Math library is duplicated in the Clib object.
The Clib object contains functions that are a part of the standard
C library. Some functions have been omitted or moved to the
`obsolete' section at the end of this chapter. This is because
their action has been taken over by the ScriptEase processor,
or they are duplicated by Javascript behavior. Obsolete
functions may be used.
.asctime(Time)
Returns a string representing the date and time extracted
from a Time object (as returned by localtime()). The string
will have this format:
Mon Jul 19 09:14:22 1993\n
Note that this function will not work with Javascript
Date objects. The Clib functions create a Time object
which is distinct from the standard Javascript Date
object. You can not use Date object properties with
a Time object or vice versa.
.clock()
Returns the current processor tick count. Clock value
starts at 0 when ScriptEase program begins and is
incremented CLOCKS_PER_SEC times per second.
.ctime(time)
This function is equivalent to: asctime(localtime(time)).
Time is a date-time value as returned by the time() function.
.difftime(time0 - time1)
This function returns the difference in seconds between two
times. Time1 and time0 are the value types as
returned by the time() function.
.gmtime(time)
Takes the integer time, as returned by the time() function, and converts it to a Time object representing the current date and time expressed as Greenwich mean time. See localtime() for a description of the returned object.
.localtime(timeInt)
This function returns the value timeInt (as returned by the time() function) as a Time object. Note that the Time object differs from the Date object, although they contain the same data. The Time object is for use with the other date and time functions in the .Clib object. It has the following integer properties:
| .tm_sec | second after the minute (from 0) |
| .tm_min | minutes after the hour (from 0) |
| .tm_hour | hour of the day (from 0) |
| .tm_mday | day of the month (from 1) |
| .tm_mon | month of the year (from 0) |
| .tm_year | years since 1900 (from 0) |
| .tm_wday | days since Sunday (from 0) |
| .tm_yday | day of the year (from 0) |
| .tm_isdst | daylight-savings-time flag
|
EXAMPLE: This function prints the current date
and time on the screen and returns the day of the year,
where Jan 1 is the 1st day of the year:
ShowToday()
// show today's date; return day of the year in USA format
{
// get current time structure
tm = localtime(time());
// display the date in USA format
printf("Date: %02d/%02d/%02d ", tm.tm_mon+1,
tm.tm_mday,
tm.tm_year % 100);
// convert hour to run from 12 to 11, not 0 to 23
hour = tm.tm_hour % 12;
if ( hour == 0 )
hour = 12;
// print current time
printf("Time: % 2d:%02d:%02d\n", hour, tm.tm_min,
tm.tm_sec);
// return day of year, where Jan. 1 would be day 1
return( tm.tm_yday + 1 );
}
.mktime(Time)
This returns the calendar time, which is the time format returned by time(), corresponding to a Time object as returned by localtime(). All undefined elements of Time will be set to 0 before the conversion. It returns -1 if time cannot be converted or represented.
.strftime( stringVar, formatString, time)
This function creates a string that describes the date and or time and stores it in the variable stringVar.
formatString
describes what the string will look like;
tm
is a time object as returned by localtime().
These following conversion characters may be used with strftime() to indicate time and date output:
| %a | abbreviated weekday name (Sun) |
| %A | full weekday name (Sunday) |
| %b | abbreviated month name (Dec) |
| %B | full month name (December) |
| %c | date and time (Dec 2 06:55:15 1979) |
| %d | two-digit day of the month (02) |
| %H | two-digit hour of the 24-hour day (06) |
| %I | two-digit hour of the 12-hour day (06) |
| %j | three-digit day of the year from 001 (335) |
| %m | two-digit month of the year from 01 (12) |
| %M | two-digit minute of the hour (55) |
| %p | AM or PM (AM) |
| %S | two-digit seconds of the minute (15) |
| %U | two-digit week of the year where Sunday is first day of the week (48) |
| %w | day of the week where Sunday is 0 (0) |
| %W | two-digit week of the year where Monday is the first day of the week (47) |
| %x | the date (Dec 2 1979) |
| %X | the time (06:55:15) |
| %y | two-digit year of the century (79) |
| %Y | the year (1979) |
| %Z | name of the time zone, if known (EST) |
| %% | the per cent character (%) |
EXAMPLE: The following code prints the full day
name and month name of the current day:
strftime(TimeBuf,"Today is: %A, and the month is: %B",
localtime(time()));
puts(TimeBuf);
.time(t)
Returns an integer representation of the current time.
The format of the time is not specifically defined except
that it represents the current time, to the system's best
approximation, and can be used in many other time-related
functions. If t is supplied then it will be set to equal
the returned value.
.assert(boolean)
If boolean evaluates to FALSE this function will print the file name and line number to stderr and abort. If the assertion evaluates to TRUE then the program continues. assert() is typically used as a debugging technique to test assumptions before executing code based on those assumptions. Unlike C, the ScriptEase implementation of assert does not depend upon NDEBUG being defined or undefined. It is always active.
EXAMPLE: The Inverse() function returns the inverse of the input number (i.e., 1/x):
Inverse(x) // return 1/x
{
assert(0 != x);
return 1 / x;
}
.atexit(string)
This function registers a function to be called when the
script ends. The string passed to this function is the name
of a function to be called.
.exit(status)
This function causes normal program termination. It calls
all functions registered with atexit(), flushes and closes
all open file streams, updates environment variables if
applicable to this version of ScriptEase, and returns control
to the OS environment with the return code of status.
.system(commandString)
(DOS versions only): .system(P_SWAP, commandString)
Passes commandString to the command processor and
returns whatever value was returned by the command processor.
CommandString may be a formatted string followed by variables
according to the rules defined in sprintf().
DOS |
In the DOS version of ScriptEase, if the special argument
P_SWAP is used then SEDOS.exe is swapped to EMS/XMS/INT15
memory or disk while the system command is executed. This
leaves almost all available memory for executing the command.
See spawn() for a discussion of P_SWAP. |
DOS32 |
The 32-bit protected mode version of DOS ignores the first
parameter if it is an not a string; in other words, P_SWAP
is ignored. |
.ferror(filePointer)
This function tests and returns the error indicator for stream file.
filePointer is a file pointer as returned by fopen().
Returns 0 if no error, otherwise returns the error value.
.errno
.perror([string])
Prints and returns an error message that describes the error
defined by errno. This function is identical to calling
strerror(errno). If string is supplied it will be set to the
value of the error string and returned.
.strerror(errno)
An error number may be generated by certain library calls,
such as fopen(). This function converts the error to a
descriptive string and returns it.
EXAMPLE: This function opens a file for reading, and if
it cannot open the file then it prints a descriptive message
and exits the program:
MustOpen(filename)
{
fh = fopen(filename,"r");
if ( fh == NULL ) {
printf("Unable to open %s for reading.\n",filename);
printf("Error:%s\n",strerror(errno));
exit(EXIT_FAILURE);
}
return(fh);
}
.fclose(fp)
fp is a file pointer as returned by fopen(). This
function flushes the stream's file buffers and closes the file.
The file pointer ceases to be valid after this call. Returns
zero for success, otherwise returns EOF.
.feof(fp)
fp is a file pointer as returned by fopen().
This function returns an integer which is non-zero
if the file cursor is at the end of the file, and 0
if it is NOT at the end of the file.
.fflush(fp)
Causes any unwritten buffered data to be written to
fp. If fp is NULL then flushes buffers in all
open files. Returns zero if successful; otherwise EOF.
.fgetc
This function returns the next character in the file stream indicated by
fp as a byte converted to an integer. If there is a read error or the file cursor is at the end of the file EOF will be returned. If there is a read error then ferror() will indicate the error condition.
.fgetpos(fp, pos)
This function stores the current position of the file
stream pointer for future restoration using fsetpos().
The file position will be stored in the variable pos;
use it with fsetpos() to restore the cursor to its
position. Returns zero for success, otherwise returns
non-zero and stores an error value in errno.
fgets(fp)
This returns a string consisting of the characters in a file from the current file cursor to the next newline character, or untill buflen-1 characters are read, whichever comes first. If a newline was read it will be returned as part of the string. If there is an error or the end of the file is reached NULL will be returned.
.fopen(filename, mode)
This function opens the file specified by filename for file
operations specified by mode, returning a file pointer (fp)
to the file opened. NULL is returned in case of failure.
Filename is a string. It may be any valid file
name, excluding wildcard characters.
Mode is a string composed of `r', `w', or `a'
followed by other characters as follows:
r | open file for reading; file must already exist |
w | open file for writing; create if doesn't exist; if file exists then truncate to zero length |
a | open file for append; create if doesn't exist; set for writing at end-of-file |
b | binary mode; if b is not specified then open file in text mode (end-of-line translation) |
t | text mode |
+ | open for update (reading and writing) |
When a file is successfully opened, its error status is
cleared and a buffer is initialized for automatic buffering
of reads and writes to the file.
EXAMPLE: The following code opens the text file
"ReadMe" for text-mode reading, and prints out
each line in that file:
fp = fopen("ReadMe","r");
if ( fp == NULL )
printf("\aError opening file for reading.\n")
else {
while ( NULL != (line=fgets(fp)) ){
fputs(line,stdout);
}
fclose(fp);
.fprintf(filePointer, formatString,...)
This flexible function writes a formatted string to the
file associated with filePointer. The second parameter,
formatString, is a string of the same pattern as
Clib.sprintf() and SElib.rsprintf.
.fputc(charVar, fp)
If charVar is a string, the first character of the
string will be written to the file indicated by fp.
If charVar is a number, the character corresponding
to its UNICODE value will be added.
If successful, the character written will be returned,
otherwise EOF is returned.
.fputs(string, filePointer)
Writes the value of string to the file indicated by
filePointer. Returns EOF if write error, else returns
a non-negative value.
.fread(DestBuffer, bufferLen, fp)
.fread( DestVar, int DataTypeInFile, fp)
.fread(struct DestStruct, DataStructureDefinition, fp)
This function reads data from a file opened for reading into a
destination variable. The destination is created, if necessary,
to contain the data read. In the first syntax form for this
routine, up to bufferLen bytes are read into DestBuffer.
In the second syntax form for this routine, a single data item
is read into DestVar; the DataTypeInFile parameter specifies how
that data is to be read from the file. DataTypeInFile may be one
of the following:
| UWORD8 | Stored as a byte in DestVar |
| SWORD8 | Stored as an integer in DestVar |
| UWORD16 | Stored as an integer in DestVar |
| SWORD16 | Stored as an integer in DestVar |
| UWORD24 | Stored as an integer in DestVar |
| SWORD24 | Stored as an integer in DestVar |
| UWORD32 | Stored as an integer in DestVar |
| SWORD32 | Stored as an integer in DestVar |
| FLOAT32 | Stored as a float in DestVar |
| FLOAT64 | Stored as a float in DestVar |
| FLOAT80 | Stored as a float in DestVar |
In the third form, which reads data into an object, the DataStructureDefinition sets each possible element of DestStruct from the same elements of DataStructureDefinition, with the exception that member names beginning with an underscore (_) are not copied. This allows you to read only those portions of the structure in the file that you are interested in. For example, the definition of a structure might be:
ClientDef.Sex = UWORD8;
ClientDef.MaritalStatus = UWORD8;
ClientDef._Unused1 = UWORD16;
ClientDef.FirstName = 30;
ClientDef.LastName = 40;
ClientDef.Initial = UWORD8;
The ScriptEase version of fread() differs from the standard
C version in that the standard C library is set up for reading
arrays of numeric values or structures into consecutive bytes
in memory.
For the second and third forms, data types will be read from
the file in a byte-order described by the current value of
the _BigEndianMode variable (see _BigEndianMode in chapter 8).
Modifies the first input parameter. If DestBuffer is the
first parameter then set buffer to be an array of characters.
If buffer is a DataType then create or modify DestVar to hold
the numeric DataType.
In all cases, this function returns the number of elements
read. For DestBuffer this would be the number of bytes read,
up to bufferLen. For DataTypeInFile this returns 1 if the
data is read or 0 if read error or end-of-file is encountered.
EXAMPLE: To read the 16-bit integer `i', the 32-bit
float `f', and then 10-byte buffer `buf' from open file `fp':
if ( !fread(i,SWORD16,fp) || !fread(f,FLOAT32,fp)
|| 10 != fread(buf,10,fp) ) {
printf("Error reading from file.\n");
abort();
}
.freopen(filename, mode, oldFp)
This function closes the file associated with OldFP (ignoring
any close errors), and then opens filename according to mode,
just as in the fopen() call, and reassociates OldFP to this
new file specification. This function is most commonly used
to redirect one of the pre-defined file handles (stdout,
stderr, stdin) to or from a file.
The function returns a copy of the modified OldFP, or NULL if it fails.
EXAMPLE: This sample code will call the ScriptEase for
DOS program with no parameters (which causes a help screen to
be printed), but redirecting stdout to a file CENVI.OUT so
that CENVI.OUT will contain the text of the ScriptEase help screens.
if ( NULL == freopen("CENVI.OUT","w",stdout) )
printf("Error redirecting stdout\a\n");
else
system("SEDOS")
.fscanf(fp, formatString [, ...])
This flexible function reads input from a file
specification and stores in parameters following
the format string according the character combinations
in the format string, which indicate how the file data
is to be read and stored. Stream must have been previously
opened with read access.
See scanf() for a description of this format string. The
parameters following the format string will be set to data
according to the specifications of the format string.
RETURN: Returns EOF if input failure before any
conversion occurs, else returns the number of input items
assigned; this number may be fewer than the number of
parameters requested if there was a matching failure.
EXAMPLE:
Given the following text file, WEIGHT.DAT:
Crow, Barney 180
Claus, Santa 306
Mouse, Mickey 2
this code:
fp = fopen("WEIGHT.DAT","r");
FormatString = "%[,] %*c %s %d\n";
while (3==fscanf(fp,FormatString,LastName, Firstame,weight) )
printf("%s %s weighs %d pounds.\n", FirstName,LastName,weight);
fclose(fp);
results in this output:
Barney Crow weighs 180 pounds.
Santa Claus weighs 306 pounds.
Mickey Mouse weighs 2 pounds.
.fseek(fp, offset [,int mode])
Set the position of the file pointer of the open file stream
fp. offset is a number indicating how many bytes the
new position will be past the starting point indicated by
mode .mode can be any of the following predefined
values:
| SEEK_CUR | seek is relative to the current position of the file |
| SEEK_END | position is relative from the end of the file |
| SEEK_SET | position is relative to the beginning of the file |
If mode is not supplied then absolute offset from the
beginning of file (SEEK_SET) is assumed. For text files (i.e.,
not opened in binary mode) the file position may not correspond
exactly to the byte offset in the file.
This function returns zero for success, or non-zero if cannot
set the file pointer to the indicated position.
.fsetpos(fp, pos)
Set the current file stream pointer to the value defined by
pos, which must be a value obtained from a previous call to
fgetpos() on the same open file. Returns zero for success,
otherwise returns non-zero and stores an error value in errno.
.ftell(fp)
Gets the position offset of the file pointer of an open file
stream from the beginning of the file. For text files (i.e.,
not opened in binary mode) the file position may not correspond
exactly to the byte offset in the file. Returns the current
value of the file position indicator, or -1 if there is an
error, in which case an error value will be stored in errno.
.fwrite(SourceBuffer, bufferLen, fp)
.fwrite(SourceVar, DataTypeInFile, fp)
Writes data from a variable into the current location of a
file opened for writing and returns the number of elements
written. For DestBuffer this would be the number of bytes
written, up to bufferLen. For DataTypeInFile this returns
1 if the data is written or 0 if a write error or end-of-file
is encountered..
In the first syntax form of this routine, up to bufferLen
bytes are written from the SourceBuffer byte array.
In the second syntax form of this routine, a single data
item is written from SourceVar, and the DataTypeInFile
parameter specifies how that data is to be read from the
file. See fread() (above) for valid DataTypeInFile parameters.
The ScriptEase version of fwrite() differs from the standard
C version in that the standard C library is set up for writing
arrays of numeric values or structures from consecutive bytes
in memory.
EXAMPLE: To write the 16-bit integer `i', the 32-bit
float `f', and then 10-byte buffer `buf' into open file `fp':
if ( !fwrite(i,SWORD16,fp) || !fwrite(f,FLOAT32,fp)
|| 10 != fwrite(buf,10,fp) )
{
printf("Error writing to file.\n");
abort();
}
.getc(fp)
This function is identical to fgetc(). It returns the
next character in the file fp as a byte (unsigned)
converted to an integer. Returns EOF if there is a
read error or if at end-of-file; if read error then
ferror() will indicate the error condition.
.remove(filename)
Deletes the file specified by filename. Returns zero if successful and non-zero for failure.
.rename(oldFilename, string newFilename)
This function renames oldFilename to newFilename. Both
oldFilename and newFilename are strings. Returns zero if
successful and non-zero for failure.
.rewind(fp)
Set the file cursor to the beginning of file. This call
is identical to fseek(stream,0,SEEK_SET) except that it
also clears the error indicator for this stream.
.tmpfile()
This returns the file pointer of a temporary binary file
that will automatically be removed when it is closed or
when the program exits. If FILE variable is an environment
variable then it is invalid after the program ends. NULL
will be returned if the function fails.
.tmpnam([string])
This creates a string that is a valid file name that is not
the same as the name of any existing file and not the same
as any filename returned by this function during execution
of this program. If a string is supplied it will be set to
the string that will be returned by this function.
.cosh(number)
Returns the hyperbolic cosine of x.
.div(numerator, denominator)
This function devides numerator by denominator and returns the result as an object with the following properties:
| .quot | quotient |
| .rem | remainder |
.frexp(x)
Breaks x into a normalized mantissa between 0.5 and 1.0, or
zero, and calculates an integer exponent of 2 such that
x=mantissa*2 ^ exponent. Exponent will be modified
to contain the appropriate value, and a normalized mantissa
between 0.5 and 1.0, or 0 will be returned.
.ldexp()
This is the inverse function of frexp().
It calculates mantissa * 2
exponent and returns the result.
.log10(x)
Returns the base-ten logarithm of x.
.modf(x, i)
This function splits x into integer and fraction parts, setting i to the integer part of x and returning the fraction part.
.sinh(x)
Returns the hyperbolic sine of x
Sorting functions:
.bsearch( key, SortedArray, [int ElementCount,] CompareFunction)
The CompareFunction will receive the key variable as its first argument and a variable from the array as its second argument. If ElementCount is not supplied then will search the entire array. ElementCount is limited to 64K for 16-bit version of ScriptEase.
RETURN: Will return the array variable at the offset in
the array of the found element, else NULL if the key is not
matched in the array. This function assumes all array indices
are greater than or equal to 0.
EXAMPLE: The following example demonstrates the use of
qsort() and bsearch() to locate a name and related item in a list:
// create array of names and favorite food
list = {
{ "Brent", "salad" },
{ "Laura", "cheese" },
{ "Alby", "sugar" },
{ "Josh", "anything" },
{ "Aiko", "cats" },
{ "Quinn", "anything from the garbage" }
};
// sort the list
qsort(list,"ListCompareFunction");
Key[0] = "brent";
// search for the name Brent in the list
Found = bsearch(Key,list,"ListCompareFunction");
// display name, or not found
if ( Found )
printf("%s's favorite food is %s\n",
Found[0][0],Found[0][1]);
else
puts("Could not find name in list.");
ListCompareFunction(Item1,Item2)
return strcmpi(Item1[0],Item2[0]);
}
.qsort(array, [ ElementCount,] CompareFunction)
Sort elements in this array, starting from index 0 to ElementCount-1. If ElementCount is not supplied then will sort the entire array. This function differs from the Array.sort() method in that it can sort automatically created arrays, whereas Array.sort() only works with arrays explicity created with a new Array statement.
ElementCount is limited to 64K
EXAMPLE: The following code would print a list of colors
sorted reverse-alphabetically and case-insensitive:
// initialize an array of colors
colors = { "yellow", "Blue", "GREEN", "purple",
"RED", "BLACK", "white", "orange" };
// sort the list using qsort and our ColorSorter routine
qsort(colors,"ReverseColorSorter");
// display the sorted colors
for ( i = 0; i <= GetArraySpan(colors); i++ )
puts(colors[i]);
ReverseColorSorter(color1,color2)
// do a simple case insensitive string
// comparison, and reverse the results too
{
CompareResult = stricmp(color1,color2)
return( -CompareResult );
}
The output of the above code would be:
yellow
white
RED
purple
orange
GREEN
Blue
BLACK
.getenv([VariableName])
If VariableName is supplied, this returns the value of a similarly named environment variable as a string if the variable exists, it returns NULL if VariableName does not exist. If no name is supplied then returns an array of all environment variable names, ending with a NULL element.
EXAMPLE: The following code would print the existing
environment variables, in "EVAR=Value" format,
sorted alphabetically.
// get array of all environment variable names
EnvList = getenv();
// sort array alphabetically
qsort(EnvList,GetArraySpan(EnvList),"stricmp");
// display each element in ENV=VALUE format
for ( lIdx = 0; EnvList[lIdx]; lIdx++ )
printf("%s=%s\n",EnvList[lIdx],getenv(EnvList[lIdx]));
.putenv(varName, stringValue)
Sets the environment variable varName to the value of
string. If string is NULL then varName is
removed from the environment. For those operating systems for
which ScriptEase can alter the parent environment (DOS, or OS/2
when invoked with SESet.cmd or using ESet()) the variable setting
will still be valid when ScriptEase exits; otherwise the variable
change applies only to the ScriptEase code and to child processes
of the ScriptEase program. Returns -1 if there is an error, else 0.
.isalnum(string)
Returns TRUE if all of the characters in string are either letters
(A to Z or a to z) or digits (0 to 9); otherwise returns FALSE.
.isalpha(string)
Returns TRUE if string consists entirely of letters
(A to Z or a to z), otherwise returns FALSE.
.isascii(string)
Returns TRUE if consists entirely of ASCII characters,
otherwise returns FALSE.
.iscntrl(string)
Returns TRUE if string contains any control characters
or delete characters. Returns FALSE if there are none.
.isdigit(string)
Returns TRUE if string consists entirely of digits (0 to 9);
otherwise returns FALSE.
.isgraph(string)
Returns TRUE if string consists entirely of printable
characters other than spaces, otherwise returns FALSE.
.islower(string)
Returns TRUE if string consists entirely of lowercase
letters (a to z); otherwise returns FALSE.
.isprint(string)
Returns TRUE if string consists entirely of printable
characters, otherwise returns FALSE.
.ispunct(string)
Returns TRUE if string contains any punctuation characters;
returns FALSE if there are no punctuation characters in the string.
.isspace(string)
Returns TRUE if string contains any whitespace characters;
returns FALSE if there are none.
.isupper(string)
Returns TRUE if string consists entirely of uppercase letters
(A to Z); otherwise returns FALSE.
.isxdigit(string)
Returns TRUE if string can be converted to a hexadecimal digit
(0 to 9, A to F, or a to f), otherwise returns FALSE.
SCANF() IS MISSING
.sprintf(string, formatString...)
This method writes output to the string variable specified by buffer according to
formatString, and returns the number of characters written into buffer or EOF if there was an error. formatString can contain character combinations indicating how following parameters may be written. string need not be previously defined; it will be created large enough to hold the result. See printf() for a description of formatString.
The format string may contain character combinations
indicating how following parameters are to be treated.
Characters are printed as read to standard output until
a per cent character (`%') is reached. % indicates that
a value is to be printed from the parameters following
the format string. Each subsequent parameter specification
takes from the next parameter in the list following format
(angled brackets represent required fields, while square
brackets represent optional fields):
%[flags][width][.precision]<type>
Where flags may be:
| - | Left justification in the field with blank padding; else right justifies with zero or blank padding |
| + | Force numbers to begin with a plus (+) or minus (-) |
| blank | Negative values begin with a minus (-); positive values begin with a blank |
| # | Convert using the following alternate form, depending on output data type |
| c, s, d, i, u | No effect. |
| o | 0 (zero) is prepended to non-zero output. |
| x, X | 0x, or 0X, are prepended to output. |
| f, e, E | Output includes decimal even if no digits follow decimal. |
| g, G | same as e or E but trailing zeros are not removed. |
width may be:
| n | (n is a number e.g., 14) At least n characters are output, padded with blanks. |
| 0n | At least n characters are output, padded on the left with zeros> |
| * | The next value in the argument list is an integer specifying the output width. |
| .precision | If precision is specified, then it must begin with a period (.), and may be as follows. |
| | .0 | For floating point type, no decimal point is output. |
| | .n | n characters or n decimal places (floating point) are output. |
| | .* | The next value in the argument list is an integer specifying the precision width. |
type may be:
| d, i | signed integer |
| u | unsigned integer |
| o | octal integer x |
| x | hexadecimal integer with 0-9 and a, b, c, d, e, f |
| X | hexadecimal integer with 0-9 and A, B, C, D, E, F |
| f | floating point of the form [-]dddd.dddd |
| e | floating point of the form [-]d.ddde+dd or [-]d.ddde-dd |
| E | floating point of the form [-]d.dddE+dd or [-]d.dddE-dd |
| g | floating point of f or e type, depending on precision |
| G | floating point of For E type, depending on precision |
| c | character (`a', `b', `8', e.g.) |
| s | string |
To include the '%' character as a character in the format
string, you must use two % together ('%%') to prevent the
computer from trying to interpret it as one of the above.
EXAMPLES:Each of the following lines shows
a sprintf example followed by the resulting string:
sprintf(testString, "I count: %d %d %d.",1,2,3)
I count: 1 2 3
a = 1;
b = 2;
sprintf(testString, "%d %d %d",a, b, a+b)
1 2 3
.strcmpi(string1, string2)
Compare the bytes of s1 against s2, case-insensitive so that
`A' is equal to `a', until there is a mismatch or reach the
terminating null byte. Returns result of comparison, which will be:
| < | 0 if s1 is less than s2 |
| = 0 | if s1 is the same as s2 |
| > | 0 if s1 is greater than s2 |
.strcspn(string, charSet))
Searches string for any of the characters in the string
charSet, and returns the offset of that character. If
no matching characters are found, it returns the length of
the string. This function is similar to strpbrk, except that
strpbrk returns the string beginning at the first character
found, while strcspn returns the offset number for that character.
EXAMPLE: The following script demonstrates the difference between strcspn and strpbrk:
string="There's more than one way to skin a cat."
rStrpbrk = strpbrk(string, "dxb8w9k!");
rStrcspn = strcspn(string, "dxb8w9k!");
printf("The string is: %s\n", string);
printf("\nstrpbrk returns a string: %s\n", rStrpbrk);
printf("\nstrcspn returns an integer: %d\n", rStrcspn);
printf("string + strcspn = %s\n", string + rStrcspn); getch();
And results in the following output:
The string is: There's more than one way to skin a cat.
strpbrk returns a string: way to skin a cat.
strcspn returns an integer: 22
string + strcspn = way to skin a cat
stricmp(string1, string2)
Compare the bytes of s1 against s2 until there is a mismatch
or reach the terminating null byte. Comparison is case-insensitive,
so `a' == `A'. Returns the result of the comparison, which will be:
| < | 0 if s1 is less than s2 |
| = 0 | if s1 is the same as s2 |
| > | 0 if s1 is greater than s2 |
.strncat(destString, sourceString, MaxLen)
Appends up to MaxLen bytes of sourceString string onto the end
of destString. Characters following a null-byte in sourceString
are not copied. The destString array is made big enough to hold
min(strlen(sourceString),MaxLen) and a terminating null byte.
The value of destString is returned.
.strncmp(string1, string2, MaxLen)
Compare up to MaxLen bytes of s1 against s2 until there is a
mismatch or reach the terminating null byte. The comparison
ends when MaxLen bytes have been compared or when a terminating
null-byte has been compared, whichever comes first. Returns result
of comparison, which will be:
| < | 0 if s1 is less than s2 |
| = 0 | if s1 is the same as s2 |
| > | 0 if s1 is greater than s2 |
.strncpy(destString, sourceString, MaxLen)
Copies min(strlen(sourceString)+1,MaxLen) bytes from sourceString to destString. If destString is not already defined then this defines it as a string. destString is null-padded if MaxLen is greater than the length of sourceString, and a null-byte is appended to destString if MaxLen bytes are copied. It is safe to copy from one part of a string to another part of the same string. Returns the value of dest; that is, a variable into the dest array based at dest[0].
.strnicmp(string1, string2, MaxLen)
This is the same as strncmp() except that the comparison
is case-insensitive, such that `a' == `A'. It is the same
as strncmpi().It returns the result of the comparison, which
will be:
| < | 0 if s1 is less than s2 |
| = 0 | if s1 is the same as s2 |
| > | 0 if s1 is greater than s2 |
.strncpy(destString, sourceString, MaxLen)
Copies min(strlen(sourceString)+1,MaxLen)
bytes from sourceString to destString.
If destString is not already defined then this
defines it as a string. destString is null-padded
if MaxLen is greater than the length of
sourceString, and a null-byte is appended to
destString if MaxLen bytes are copied. It is
safe to copy from one part of a string to another part of
the same string. Returns the value of dest; that is, a
variable into the dest array based at dest[0].
.strpbrk(string, charSet)
Searches string string for any of the characters in
charSet, and returns the string based at the found
character. Returns NULL if no character from
charSet is found.
EXAMPLE: See strcspn() for an example using this function.
.strspn(string, charSet)
Searches string Str for any characters that are not in
charSet, and returns the offset of the first instance of
such a character. If all characters in string are also
in charSet will return the length of string.
.strstri(string, substring)
Searches string, starting at Str[0], for the first
occurrence of subString. Comparison is case-sensitive.
This is a case-insensitive version of the String.substring()
method. Returns NULL if subString is not found
anywhere in string; otherwise returns variable for
the string array based at the first offset matching
subString.
.strtok(sourceString, delimiterString)
This is a weird function.
sourceString is a string that consists of text tokens
(substrings) separated by delimiters found in delimiterString.
Bytes of sourceString may be altered during the first and
subsequent calls to strtok().
On the first call to strtok(), sourceString points
to the string to tokenize and delimiterString
is a list of characters which are used to separate
tokens in source. This first call returns a variable
pointing to the sourceString array and based
at the first character of the first token in
sourceString. On subsequent calls, the first
argument is NULL and strtok will continue through
sourceString returning subsequent tokens.
The ScriptEase implementation of this function is as weird
as any: the initial var must remain valid throughout following
calls with NULL as the initial var. If you change the string
in any way, a following call to strtok() must be of the first
syntax form (i.e., the new string must be passed as a first
parameter). Returns NULL if there are no more tokens; otherwise
returns sourceString array variable based at the next
token in sourceString.
EXAMPLE: This code:
source = " Little John,,,Eats ?? crackers;;;! ";
token = strtok(source,", ");
while( NULL != token ) {
puts(token);
token = strtok(NULL,";?, "); }
produces this list of tokens:
Little
John
Eats
crackers
!
.tanh(x)
Returns the hyperbolic tangent of x.
.va_arg()
.va_arg(int offset)
.va_arg(blob valist[, int offset])
va_arg() is called to retrieve a parameter passed to a
function when the number of parameters passed to the function
is not constant. This function covers the same territory as the
Function.arguments[] property and is provided for backwards
compatibility with earlier versions of ScriptEase and as an
alternate way to handle functions with a variable number of
arguments.
The va_arg() form of this function returns only the number of
parameters passed to the current function.
If the va_arg(int offset) form is used then this returns the
input variable at index: offset.
If valist is used then the variable returned is the one at
offset from the first of the valist variables. The valist
must be initialized with a call to va_start before you can
call va_arg() with this syntax. If offset is not passed in
then the next variable from valist is retrieved and valist
is updated so that a further call to va_arg(valist) will
return the subsequent variable.
It is a fatal error to retrieve an argument offset beyond
the number of parameters in the function or the valist.
.va_start(va_list[, InputVar])
This function initializes va_list for a function that takes
a variable number of arguments. After this call, va_list will
be a BLOb that may then be used in further calls to va_arg()
to get the next argument(s) passed to the function.
InputVar must be one of the parameters defined
in the function line; the first argument returned by
the first call to va_arg() will be the variable passed
after InputVar. If InputVar is not provided, then the
first parameter passed to the function will be the first
one returned by va_arg(va_list).
Returns the number of valid calls to va_arg(va_list),
i.e., how many variables are available in this va_list.
EXAMPLE: The following example uses and accepts
a variable number of strings and concatenates them all together:
MultiStrcat(Result,InitialString)
// Append any number of strings to InitialString.
// e.g., MultiStrcat(Result,"C:\\","FOO",".","CMD")
{
strcpy(Result,""); // initialize the result
Count = va_start( ArgList, InitialString )
for ( i = 0; i < Count; i++ )
strcat( Result, va_arg(ArgList) )
}
.vfprintf(filePointer, formatString, ...)
This function formats a string with a variable number
of arguments and prints it to the file specified by
filePointer. It returns the number of characters written,
or a negative number if there was an output error. See
fprintf() and sprintf() for more details.
.vfscanf(filePointer, formatString, ...)
This is similar to fscanf() except that it takes a variable
argument list (see va_start()). See fscanf() for more details.
.vscanf(string format, blob valist)
This is similar to scanf() except that it takes a variable
argument list (see va_start()). The parameters following
the format string will be assigned values according to the
specifications of the format string. See scanf() for more details.
Returns the number of input items assigned; this number may
be fewer than the number of parameters requested if there
was a matching failure.
EXAMPLE: The following function acts like scanf,
taking a variable number of input arguments, except it
beeps and tries again if there are no matches:
Must_scanf(FormatString /*,arg1,arg2,arg3,etc...*/ )
{
vastart(va_list,FormatString);
// create variable arg list
do { // mimic original scanf() call
count = vscanf(FormatString,va_list);
if ( 0 == count ) // if no match, then beep
printf("\a");
} while( 0 == count );
// if not match, then try again
return(count);
}
.vsprintf(string buffer,string format, blob valist)
This is similar to sprintf() except that it takes a variable
argument list (see va_start()). It returns the number of
characters written into buffer not including the terminating
null byte, or EOF if there was an error. See sprintf() for
more details.
.vsscanf(string buffer, string format, blob valist)
This is similar to sscanf() except that it takes a variable
argument list (see va_start()). The parameters following the
format string will be assigned values according to the
specifications of the format string.
The function returns the number of input items assigned; this
number may be fewer than the number of parameters requested
if there was a matching failure. See sscanf() for more details.
|